This function block from run in task Back, manages communication with UDP protocol in client mode. It is necessary to define in PeerAdd the IP address and in PeerPort the UDP port of the server system to which you want to connect.
Activating the command Connect the connection with the server system is opened, in the UDP connection it is not possible to verify if the server is present and accepts the connection, therefore it is always activated Connected and on the exit File the stream to be used for data exchange with the server system is returned.
In LocalAdd e LocalPort you can define the IP address and port of the network interface from which to connect. If the connection is not possible it is generated Fault.
Function lock
CODESYS: Not available
LogicLab: eLLabXUnified12Lib
Description
Connect (BOOL) Connection enabling command.
PeerAdd (@STRING) IP address of the server system to connect to.
PeerPort (UINT) UDP port number to connect to.
LocalAdd (@STRING) IP address of the network interface from which to connect. Default '0.0.0.0': the interface is automatically chosen based on the IP to connect to.
LocalPort UINT) UDP port number from which the connection starts (0 chosen automatically).
FlushTm (UINT)) Data flush time (mS). If no data is loaded on the stream after the defined time, the data present are automatically sent.
LifeTm (UINT) Socket life time (S), if no data is received or sent after the defined time the socket is automatically closed. If defined time "0" the socket is never closed.
RxSize (UINT) Data reception buffer size.
TxSize (UINT) Data transmission buffer size.
Connected (BOOL) Active if connection established with server.
Fault (BOOL) Active if management error.
File (FILEP) I/O stream valued on connection established with the server system. If the connection is not active, NULL is returned
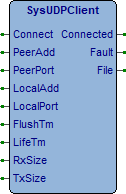
Examples
How to use the examples.
FBD_SysUDPClient, ST_SysUDPClient: In the example a connection to a UCP server listening on port 1000 is activated. Once the connection is made, the characters received from the server are sent back in echo. The local port is automatically chosen by the system.
SyslogClient: Implements a Syslog client with sending messages to servers.
LogicLab (Ptp116, FBD_SysUDPClient)
PROGRAM FBD_SysUDPClient
VAR
Fp : eFILEP; (* File pointer *)
UDPClient : SysUDPClient; (* UDP client *)
END_VAR
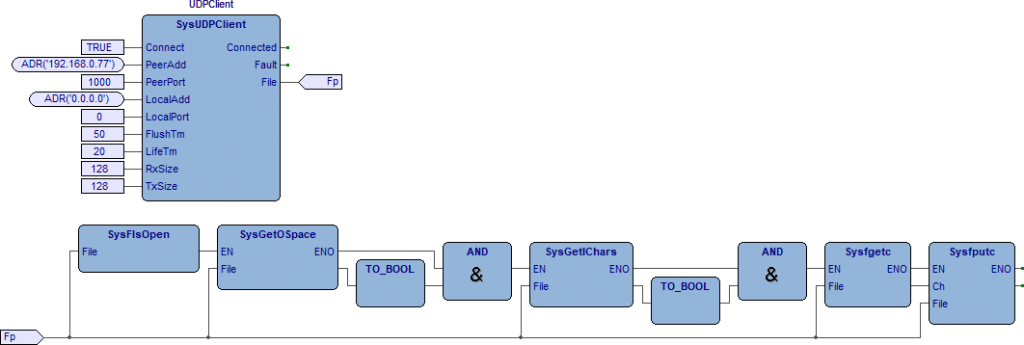
LogicLab (Ptp116, ST_SysUDPClient)
PROGRAM ST_SysUDPClient
VAR
i : UDINT; (* Auxiliary variable *)
Fp : eFILEP; (* File pointer *)
UDPClient : SysUDPClient; (* UDP client *)
END_VAR
// *****************************************************************************
// PROGRAM "FBD_SysUDPClient"
// *****************************************************************************
// A UDP client is instantiated, it connects to the UDP server IP and port
// defined. Data received are echoed.
// -----------------------------------------------------------------------------
// -------------------------------------------------------------------------
// INITIALIZATION
// -------------------------------------------------------------------------
// First program execution loop initializations.
IF (SysFirstLoop) THEN
UDPClient.PeerAdd:=ADR('192.168.0.77'); //Peer address
UDPClient.PeerPort:=1000; //Peer port
UDPClient.LocalAdd:=ADR('0.0.0.0'); //Local address
UDPClient.LocalPort:=0; //Local port
UDPClient.FlushTm:=50; //Flush time (mS)
UDPClient.LifeTm:=30; //Life time (S)
UDPClient.RxSize:=128; //Rx buffer size
UDPClient.TxSize:=128; //Tx buffer size
END_IF;
// Manage the UDP client.
UDPClient(Connect:=TRUE); //TCPClient management
Fp:=UDPClient.File; //File pointer
// -------------------------------------------------------------------------
// ECHO LOOP
// -------------------------------------------------------------------------
// Execute the echo loop.
IF (SysFIsOpen(Fp)) THEN
IF (TO_BOOL(SysFGetIChars(Fp)) AND TO_BOOL(SysFGetOSpace(Fp))) THEN
i:=Sysfputc(Sysfgetc(Fp), Fp); //Character echo
END_IF;
END_IF;
// [End of file]
LogicLab (SyslogClient
)
PROGRAM SyslogClient
VAR
Cmd : BOOL; (* Send command *)
i : UDINT; (* Auxiliary variable *)
Facility : USINT; (* Facility number *)
Severity : USINT; (* Severity number *)
CaseNr : USINT; (* Program case *)
Months : @STRING; (* Month definitions *)
UDPClient : SysUDPClient; (* UDP client *)
DateTime : SysETimeToDate; (* Date time conversion *)
END_VAR
// *****************************************************************************
// PROGRAM "SyslogClient"
// *****************************************************************************
// This program forward information as syslog messages to a syslog server.
// -----------------------------------------------------------------------------
// -------------------------------------------------------------------------
// INITIALIZATION
// -------------------------------------------------------------------------
// First program execution loop initializations.
IF (SysFirstLoop) THEN
UDPClient.PeerAdd:=ADR('192.168.0.184'); //Peer address
UDPClient.PeerPort:=514; //Peer port
UDPClient.LocalAdd:=ADR('0.0.0.0'); //Local address
UDPClient.LocalPort:=0; //Local port
UDPClient.FlushTm:=50; //Flush time (mS)
UDPClient.LifeTm:=30; //Life time (S)
UDPClient.RxSize:=128; //Rx buffer size
UDPClient.TxSize:=512; //Tx buffer size
END_IF;
// -------------------------------------------------------------------------
// SENDING SEQUENCES
// -------------------------------------------------------------------------
// Manages the UDP Client and sending sequences.
UDPClient(); //UDPClient management
CASE (CaseNr) OF
// ---------------------------------------------------------------------
// Wait command.
0:
UDPClient.Connect:=FALSE; //TCPClient management
IF NOT(Cmd) THEN RETURN; END_IF;
Cmd:=FALSE; //Send command
CaseNr:=CaseNr+1; //Program case
// ---------------------------------------------------------------------
// Connect to Syslog server.
1:
UDPClient.Connect:=TRUE; //TCPClient management
IF NOT(SysFIsOpen(UDPClient.File)) THEN RETURN; END_IF;
// ---------------------------------------------------------------------
// SEND THE MESSAGE TO SERVER
// ---------------------------------------------------------------------
// Send the message to server, here an message example.
// <19>Feb 26 17:36:42 SlimLine Prova messaggio
// ---------------------------------------------------------[PRI Part]--
// The Priority value is calculated by first multiplying the Facility
// number by 8 and then adding the numerical value of the Severity.
i:=(Facility*8)+Severity; //Priority value
i:=SysVfprintf(UDPClient.File, ADR('<%u>'), UDINT_TYPE, ADR(i));
// ------------------------------------------------------[HEADER Part]--
// Contains two fields called the TIMESTAMP and HOSTNAME.
Months:=ADR('JanFebMarAprMayJunJulAugSepOctNovDec');
DateTime(EpochTime:=SysDateTime); //Date time conversion
i:=SysVfprintf(UDPClient.File, ADR('%.3s '), STRING_TYPE, Months+(DateTime.Month*3));
i:=SysVfprintf(UDPClient.File, ADR('%2u '), USINT_TYPE, ADR(DateTime.Day));
i:=SysVfprintf(UDPClient.File, ADR('%02u:'), USINT_TYPE, ADR(DateTime.Hour));
i:=SysVfprintf(UDPClient.File, ADR('%02u:'), USINT_TYPE, ADR(DateTime.Minute));
i:=SysVfprintf(UDPClient.File, ADR('%02u '), USINT_TYPE, ADR(DateTime.Second));
i:=SysVfprintf(UDPClient.File, ADR('%s '), STRING_TYPE, ADR('SlimLine'));
// ---------------------------------------------------------[MSG Part]--
// The CONTENT contains the details of the message.
i:=SysVfprintf(UDPClient.File, ADR('%s'), STRING_TYPE, ADR('This is a message'));
// Eseguo invio messaggio di Log.
i:=SysFOBfFlush(UDPClient.File); //Flush output characters
CaseNr:=CaseNr+1; //Program case
// ---------------------------------------------------------------------
// Waits until the message is sent.
2:
IF (TO_INT(SysFGetOBfSize(UDPClient.File)) <> SysFGetOSpace(UDPClient.File)) THEN RETURN; END_IF;
CaseNr:=0; //Program case
END_CASE;
// [End of file]